Rock, Paper, Scissors
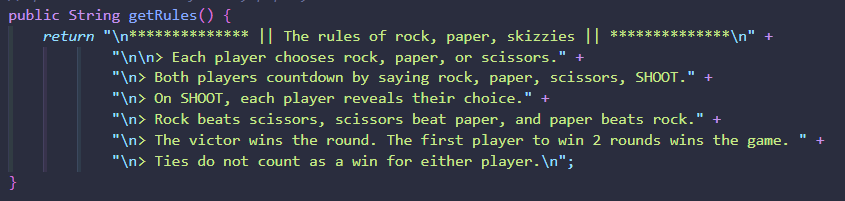
Goal
Implement a simple text-based version of the classic game "Rock, Paper, Scissors" for two players demonstrating key concepts of object-oriented programming (OOP) while providing a fun way to engage users.
How it's Accomplished
Setup: The game allows two players to compete, either against each other or with computer opponents. Each player can choose between "rock," "paper," or "scissors."
Rules: Clearly defines the rules of the game, explaining how the winner is determined and what it takes to win rounds.
Logic: Tracks the score for each player and determines when the game is over (when a player wins two rounds).
Interaction: Prompts players for their moves and reports the game state, including scores and the winner.
Techniques Used
Classes and Inheritance: The RockPaperScissors class extends TwoPlayerGame, meaning it inherits properties and methods from this parent class, allowing for shared functionality.
Encapsulation: The game's state (such as the scores and the number of rounds) is stored in private variables. This prevents direct access from outside the class, protecting the game's internal logic.
Methods: Methods like getRules(), reportGameState(), and play() break down functionality into manageable pieces. Each method has a specific role, making the code easier to read and maintain.
Polymorphism: The play method can handle both human and computer players. By using interfaces (like CLUIPlayableGame), the code can be flexible, allowing different types of players to interact with the game without changing its core logic.
Abstraction: Hides complex logic (like determining the winner) behind simple method calls. Users interact with the game through straightforward prompts and outputs, without needing to understand the underlying implementation.
Sample Output: CPU vs CPU
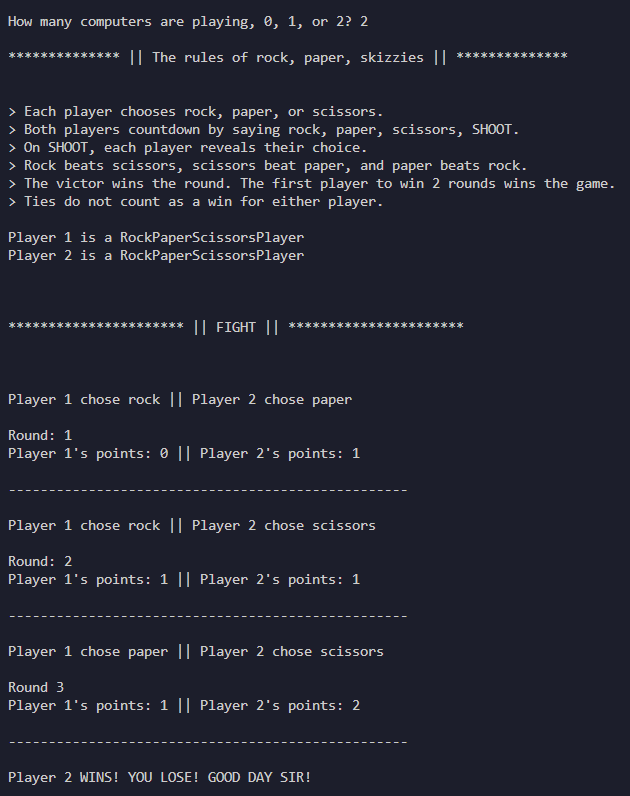